In ASP.NET Core 9, a new feature called AdditionalAuthorizationParameters allows you to customize OAuth and OpenID Connect (OIDC) flows more quickly. This new feature allows developers to add custom authentication parameters without needing to rely on the complex workarounds that existed before ASP.NET Core 9 was released. Sounds familiar? Then youāre going to like this!
In this blog, I will practically introduce you to this feature, how it works, and how it ties together with Pushed Authorization Requests and AuthenticationProperties. Letās jump in.
Why do we need AdditionalAuthorizationParameters?
Before ASP.NET Core 9, adding custom parameters to OAuth or OpenID Connect (OIDC) authorization requests was a cumbersome challenge. Developers had to override methods like BuildChallengeUrl in custom OAuth handlers or insert parameters manually using event hooks such as OnRedirectToIdentityProvider. This approach was verbose and error-prone, often leading to inconsistent project implementations.
AdditionalAuthorizationParameters solves this by providing a more straightforward and maintainable approach by including custom parameters directly in the authorization request configuration. For developers, this can be something of a godsend because it makes the code cleaner and more straightforward.
What Does Adding Custom Parameters Mean?
Customizing the initial authorization request sent to an OIDC authorization server often involves adding extra parameters to control or enhance the authentication process. A typical authorization request might look like this:
GET https://identityservice.secure.nu/connect/authorize
?client_id=localhost-addoidc-client
&redirect_uri=https%3A%2F%2Flocalhost%3A5001%2Fsignin-oidc
&response_type=code
&scope=openid%20profile%20email%20offline_access
&code_challenge=dtaF1NHSE5-C6E9eDuf-kEZJ_j7n48BaSTmezd4Go7Y
&code_challenge_method=S256
&response_mode=form_post
&nonce=638645227425598693.OGFkYjhmMWUtZ...
&state=CfDJ8IgPXRNAZH1EkNA0dd3_JvsHlnov...
&x-client-SKU=ID_NET9_0
&x-client-ver=8.1.2.0 HTTP/1.1
...
Sometimes, you may need to add extra parameters to further customize the authentication flow, for example, adding an audience, a tenant ID, or setting a specific prompt behavior.
Are you curious about what state and nonce parameters contain?
See my blog Demystifying OpenID Connectās State and Nonce Parameters in ASP.NET Core for more details.
The Old Way: Complex Workarounds
Before .NET 9, adding custom parameters often forced developers to resort to more convoluted methods, such as:
.AddOpenIdConnect(options =>
{
...
options.Events.OnRedirectToIdentityProvider = context =>
{
context.ProtocolMessage.SetParameter("parameter1", "value1");
context.ProtocolMessage.SetParameter("parameter2", "value2");
context.ProtocolMessage.SetParameter("parameter3", "value3");
return Task.CompletedTask;
};
});
This results in an authorization request like:
GET https://identityservice.secure.nu/connect/authorize
?client_id=localhost-addoidc-client
&redirect_uri=https%3A%2F%2Flocalhost%3A5001%2Fsignin-oidc
&response_type=code
&scope=openid%20profile%20email%20offline_access
&code_challenge=dtaF1NHSE5-C6E9eDuf-kEZJ_j7n48BaSTmezd4Go7Y
&code_challenge_method=S256
&response_mode=form_post
&nonce=638645227425598693.OGFkYjhmMWUtZ...
¶meter1=value1
¶meter2=value2
¶meter3=value3
&state=CfDJ8IgPXRNAZH1EkNA0dd3_JvsHlnov...
&x-client-SKU=ID_NET9_0
&x-client-ver=8.1.2.0 HTTP/1.1
...
While this approach worked, it required extra boilerplate and was far from intuitive.
How Does AdditionalAuthorizationParameters Improve This?
In ASP.NET Core 9, this process is significantly simplified. The new AdditionalAuthorizationParameters property, added to OpenIdConnectOptions, allows developers to configure additional parameters with minimal effort:
public class OpenIdConnectOptions : RemoteAuthenticationOptions
{
...
///
/// Gets the additional parameters that will be included in the authorization request.
///
///
/// The additional parameters can be used to customize the authorization request,
/// providing extra information or fulfilling specific requirements of the
/// OpenIdConnect provider.
/// These parameters are typically, but not always, appended to the query string.
///
public IDictionary AdditionalAuthorizationParameters { get; } =
new Dictionary();
...
}
This allows you to achieve the same goal with cleaner and more readable code:
.AddMyOpenIdConnect(options =>
{
...
options.AdditionalAuthorizationParameters.Add("parameter1", "value1");
options.AdditionalAuthorizationParameters.Add("parameter2", "value2");
options.AdditionalAuthorizationParameters.Add("parameter3", "value3");
});
This is a much-needed improvement when configuring OpenID Connect, making the code more maintainable.
Upskill With Me: Courses, Workshops & Training
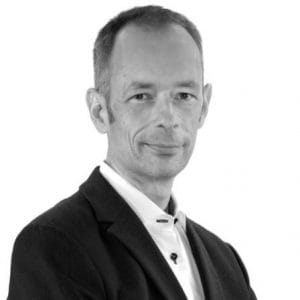
What About Pushed Authorization Requests (PAR)?
The AdditionalAuthorizationParameters feature is also compatible with Pushed Authorization Requests (PAR). When using PAR, the authorization request with AdditionalAuthorizationParameters might look like this:
POST https://identityservice.nu/connect/par HTTP/1.1
Host: identityservice.secure.nu
User-Agent: Microsoft ASP.NET Core OpenIdConnect handler
Content-Type: application/x-www-form-urlencoded
client_id=localhost-addoidc-client
&redirect_uri=https%3A%2F%2Flocalhost%3A5001%2Fsignin-oidc
&response_type=code
&scope=openid+profile+email+offline_access
&code_challenge=oIDRmjhH7UJXLaGXFNxbU5obKYXk8lMfj-OSsP73DmQ
&code_challenge_method=S256
&response_mode=form_post
&nonce=638645236162573853jIyMzc5...
¶meter1=value1
¶meter2=value2
¶meter3=value3
&state=CfDJ8IgPXRNAZH1EkNA0dd3_...
&client_secret=mysecret
As shown above, the AdditionalAuthorizationParameters are seamlessly included in the PAR request, making it compatible with modern authorization flows.
Are you curious about Pushed Authorization Requests (PAR)?
See my blog Pushed Authorization Requests (PAR) in ASP.NET Core 9 for more details.
AdditionalAuthorizationParameters vs. AuthenticationProperties
While AdditionalAuthorizationParameters allows you to easily append custom parameters to the initial OpenID Connect authorization request, AuthenticationProperties serves a different purpose.
It focuses on handling transient data stored alongside the authentication flow, such as redirection URLs or other metadata relevant to the sign-in process. In an upcoming blog post, we will explore AuthenticationProperties further.
Summary
- This improvement simplifies the customization of the initial authorization request.
- It is only applicable to the OpenID Connect authentication handler.
- It is compatible with Pushed Authorization Requests (PAR), as explained in the Pushed Authorization Requests (PAR) in ASP.NET Core 9 blog post.
Feedback, comments, found any bugs?
Let me know if you have any feedback, If I missed anything, or if there are any bugs/typos. You can find my contact details here.
About the author
Hey! I’m Tore š I’m an independent consultant, coach, trainer and a Microsoft certified MVP in .NET. My mission is to help developer teams to solve problems more quickly and effectively across tools and platforms like ASP.NET Core, Duende IdentityServer, web security, C#, Azure, .NET, and more. Sounds helpful? I’d love to be of service! You can check out my workshops for teams and my wider consulting and coaching services here.Ā
Resources
ā API Proposal – Add AdditionalAuthorizationParameters to OAuthOptions/OpenIdConnectOptions.